38. Count and Say
1. Questions
The count-and-say sequence is a sequence of digit strings defined by the recursive formula:
countAndSay(1) = "1"
countAndSay(n)
is the way you would "say" the digit string fromcountAndSay(n-1)
, which is then converted into a different digit string.
To determine how you "say" a digit string, split it into the minimal number of groups so that each group is a contiguous section all of the same character. Then for each group, say the number of characters, then say the character. To convert the saying into a digit string, replace the counts with a number and concatenate every saying.
For example, the saying and conversion for digit string "3322251"
:
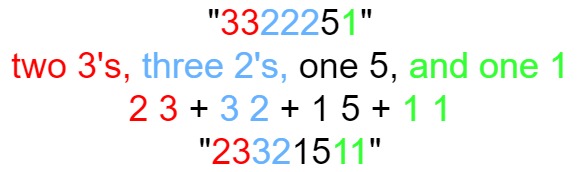
Given a positive integer n
, return the nth term of the count-and-say sequence.
2. Examples
Example 1:
Input: n = 1
Output: "1"
Explanation: This is the base case.
Example 2:
Input: n = 4
Output: "1211"
Explanation:
countAndSay(1) = "1"
countAndSay(2) = say "1" = one 1 = "11"
countAndSay(3) = say "11" = two 1's = "21"
countAndSay(4) = say "21" = one 2 + one 1 = "12" + "11" = "1211"
3. Constraints
1 <= n <= 30
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/count-and-say 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
主要采用双指针法。
right的索引刚好是str下一个循环的开始。
class Solution {
public String countAndSay(int n) {
String str = "1";
for (int i = 1; i < n; i++) {
StringBuffer sb = new StringBuffer();
int left = 0;
int right = 0;
while(right < str.length()) {
while (right < str.length() && str.charAt(right) == str.charAt(left)) {
right++;
}
sb.append(right - left).append(str.charAt(left));
left = right;
}
str = sb.toString();
}
return str;
}
}